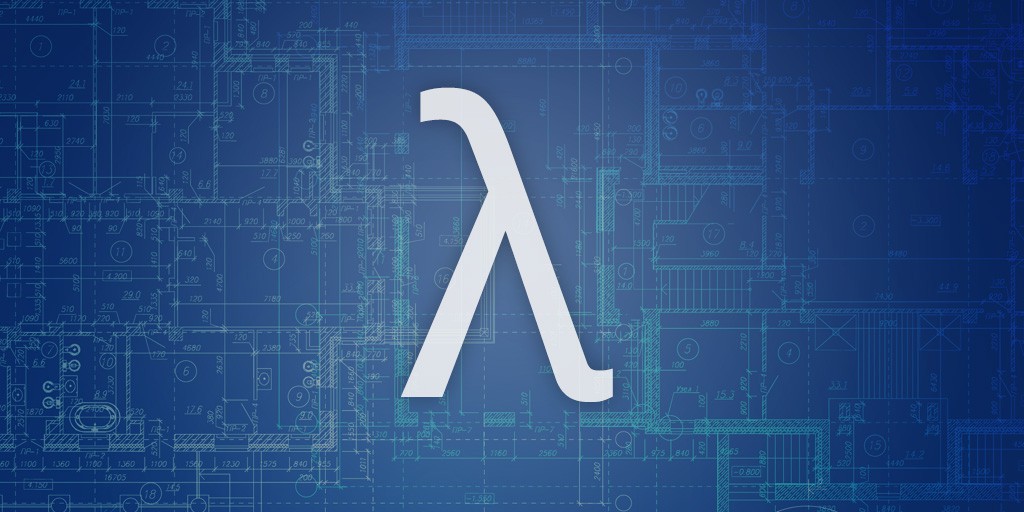
Imagine you have a task that you want to perform in your code, like adding two numbers together. In traditional programming, you would typically create a separate function or method that takes two numbers as input, performs the addition, and returns the result.
Now, with lambda expressions, you can express this task directly in your code without the need to define a separate function. It’s like having a mini-function that you can create on-the-fly, right where you need it. Lets dive into it!!!
Lambda expressions are a powerful feature introduced in Java 8 that brought functional programming capabilities to the language. They provide a concise and expressive way to represent anonymous functions, enabling developers to write more compact and readable code. In this article, we will explore the concept of lambda expressions, discuss scenarios where they shine, caution against their misuse, and provide an example to illustrate their usage.
A lambda expression is essentially a function without a name, defined as a block of code that can be passed around and executed later. It consists of parameters, an arrow operator (“->”), and a body. Lambda expressions allow you to write functional-style code by treating functions as first-class citizens.
Does the above definition confuse you? What is functional style code and first class citizen? Don’t worry, we’ll simplify it.
In functional programming, functions are treated as first-class citizens, which means they can be treated just like any other data type. They can be assigned to variables, passed as arguments to other functions, and returned as results from functions. This paradigm allows for more modular, reusable, and concise code.
Lambda expressions in Java enable the implementation of functional programming concepts by providing a way to define and use functions as objects. With lambda expressions, you can express behavior directly in your code without the need to define separate classes or interfaces. They allow you to write functions inline, right at the point of use, making the code more compact and focused.
The simplest lambda expression contains a single parameter and an expression:
parameter -> expression
Example
Filtering a List with Lambda Expressions -> Let’s consider an example where we have a list of integers and want to filter out the even numbers. Instead of using a traditional for loop or an anonymous class, we can use lambda expressions to achieve the same result more concisely:
List<Integer> numbers = Arrays.asList(11, 22, 33, 44, 55, 66);
// Filtering even numbers using lambda expression
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [22, 44, 66]
In this example, the lambda expression n -> n % 2 == 0
is passed as an argument to the filter
method. It filters out the even numbers from the list, resulting in a new list containing only the even integers.
It is very important to know when to use it and when to, here we go with this detail.
When to Use Lambda Expressions:
- Functional Interfaces: Lambda expressions are most commonly used in conjunction with functional interfaces. Functional interfaces define a single abstract method and serve as a blueprint for lambda expressions. Use lambda expressions when you need to implement the abstract method of a functional interface concisely.
- Stream Operations: Lambda expressions are extensively used in stream operations, which enable efficient processing of collections. Stream methods like
map
,filter
, andreduce
often take lambda expressions as arguments to specify the desired behavior for each element in the stream. - Event Handling: Lambda expressions provide a concise way to handle events in graphical user interfaces. Instead of implementing lengthy event listener interfaces, you can use lambda expressions to define the desired action directly.
When Not to Use Lambda Expressions:
- Complex Logic: Lambda expressions are ideal for representing simple and concise functions. If the logic becomes too complex, it may be more readable to use a traditional method with a meaningful name instead of a lambda expression.
- Code Reusability: If a piece of code needs to be reused in multiple places, it is better to define a named method. Lambda expressions are anonymous and cannot be reused across different contexts.
Conclusion
Lambda expressions are a valuable addition to Java, providing a concise and expressive way to write functional-style code. They are best utilized in scenarios involving functional interfaces, stream operations, and event handling. However, they should be used judiciously, avoiding complex logic and prioritizing code reusability. By understanding when to use and when not to use lambda expressions, developers can leverage their benefits and write cleaner and more efficient code.